Qt Signal Slot Base Class
The following sources have been used in making this material:
The QObject class is the base class of all Qt objects. QObject is the heart of the Qt ObjectModel. The central feature in this model is a very powerfulmechanism for seamless object communication called signals and slots. Youcan connect a signal to a slot with connect and destroy the connectionwith disconnect. Background:I defined a QT parent class, which has a custom signal and slot function. I defined the parent class pointer in the main function to point to the subclass object. At this time, I sent a signal, and the subclass could not receive it. We started our exploration Solution:According to the breakpoint, it is found that the signal of the. The Qt's meta-object system in Qt enables among other things signals and slots. It is based on three things: # The QObject class as the a base class for objects that can take advantage of the meta-object system.
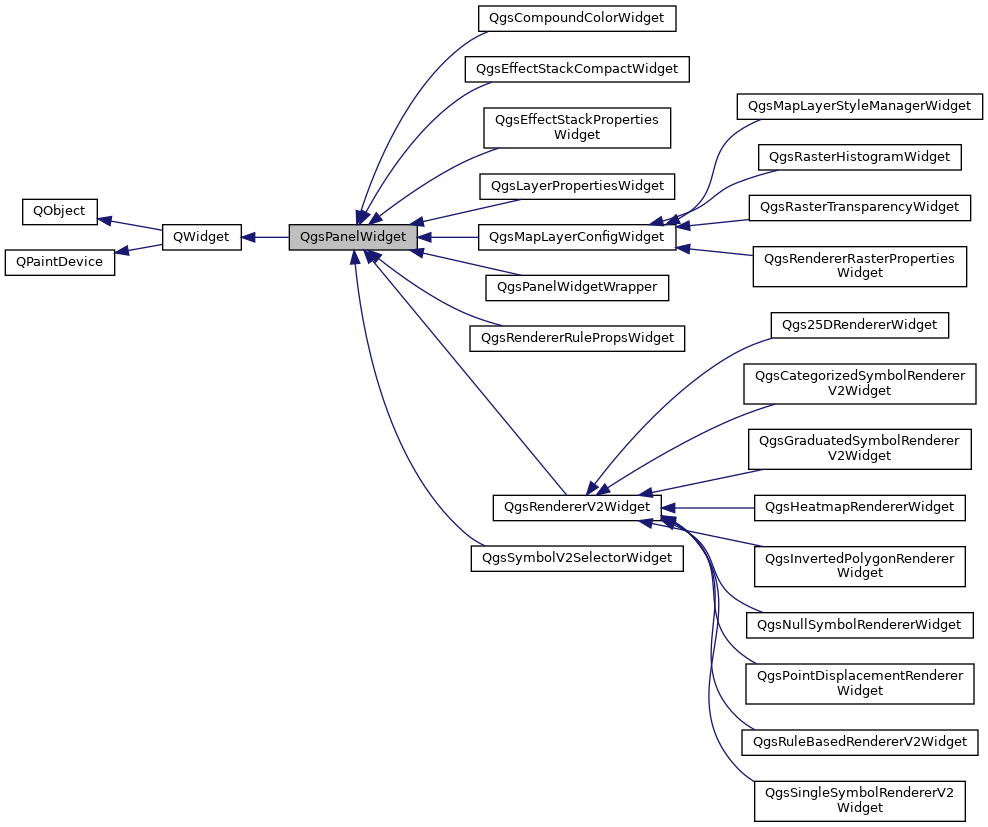
If your knowledge on signals and slots is rusty or they are a completely new concept to you, you can get started with the Programming 2 material on Qt basics.
Signals and slots¶
In Qt, signals and slots are a way for objects to communicate with eachother. A signal is emitted when some event occurs in the program. For example, when a button on the UI is pushed, the button (a QPushButton
widget) notifys the other widgets and objects about this by emitting a signal (emitclicked()
). A slot is a function called as a response to an emitted signal. For example, a slot findClicked()
is executed as a response to a button click.
All subclasses of QObject
or one of its subclasses can contain signals and slots. In practice, two things are required for the signal and slot mechanism to work. All classes that contain signals or slots must:
- mention the
Q_OBJECT
macro at the top of their declaration. - be derived from
QObject
Objects emit signals when their state changes in a way that may be interesting to other objects. The objects do not need to know anything about eachother. In fact, the only thing the object does is emit the signal. It does not know, care or need to know, if anyone receives the signals emitted and vice versa, a slot does not know if any signals are connected to it. Qt's signal and slot mechanism makes sure that if the signal is connected to a slot, the slot is automatically called with the signal's parameters.
One signal can be connected to as many slots as needed. If more than one slot is connected, they are called when the signal is emitted one after another in the same order they were connected. Similarly, more than one signal can be connected to the same slot. The slot is called when any of the signals are emitted. A signal can even be connected to another signal to emit the second signal as the first is emitted.
Signals¶
Qt's widgets have predefined signals. New signals can be defined in the class's signals
section of the interface.
Signals must not be implemented. The meta-object compiler moc generates them automatically. A signal can never have a return type and is always void
.
Slots¶
Slots are C++ functions that can be called normally as any other function. They can also be virtual if needed. In addition, signals can be connected to them. New slots are defined in the class's slots
section of the interface.
Qt Connect Signal Slot
The programmer implements the slots (slots are C++ functions). Similarly to functions, slots can be either public or private.
Qt Signal Slot Base Classic
Connecting¶
Signals and slots are connected with QObject::connect()
. The preferred syntax is functor based i.e.
where sender
is a pointer to the QObject
object emitting the signal and receiver
is a pointer to the QObject
object containing the slot. The second and fourth parameters are the signal and the slot respectively. The biggest benefits with this syntax is the compile-time type checking and the possibility to use lambdas as a part of the connect.
The other way to connect a signal to a slot is to use the SIGNAL and SLOT macros. This is typically referred as the old syntax.
where the SIGNAL and SLOT macros convert their parameters to strings. Type checking is left as a run time operation. However, you can connect C++ functions to QML function with this syntax.
Meta-object System¶
The Qt's meta-object system in Qt enables among other things signals and slots. It is based on three things:

# The QObject
class as the a base class for objects that can take advantage of the meta-object system.# The Q_OBJECT
macro at the beginning of the class declaration is used to enable meta-object features.# The Meta-Object Compiler (moc) supplies each of the QObject
subclasses with the necessary code to implement meta-object features. The moc tool reads a C++ source file. For the classes that contain the Q_OBJECTmacro
, it produces another C++ source file containing the meta-object code for each class. This generated source file is then typically compiled and linked with the class's implementation.
It is possible to use QObject
as a base class without the Q_OBJECT
macro. This means that signals, slots and the other meta-object features will not be available. For the meta-object system, a QObject
subclass without meta code is equivalent to its closest ancestor with meta-object code. Therefore, all subclasses of QObject should use the Q_OBJECT
macro even if they do not use signals, slots, and other meta-object system properties.
How often is a an object copied, if it is emitted by a signal as a const reference and received by a slot as a const reference? How does the behaviour differ for direct and queued signal-slot connections? What changes if we emit the object by value or receive it by value?
Nearly every customer asks this question at some point in a project. The Qt documentation doesn’t say a word about it. There is a good discussion on stackoverflow, which unfortunately leaves it to the reader to pick the right answer from all the answers and comments. So, let’s have a systematic and detailed look at how arguments are passed to signals and slots.
Setting the Stage
For our experiments, we need a copyable class that we will pass by const reference or by value to signals and slots. The class – let’s call it Copy
– looks as follows.
The copy constructor and the assignment operator simply perform a member-wise copy – like the compiler generated versions would do. We implement them explicitly to set breakpoints or to print debugging messages. The default constructor is only required for queued connections. We’ll learn the reason later.
We need another class, MainView
, which ultimately derives from QObject
. MainView provides the following signals and slots.
MainView
provides four signal-slot connections for each connection type.
The above code is used for direct connections. For queued connections, we comment out the first line and uncomment the second and third line.
The code for emitting the signals looks as follows:
Direct Connections
sendConstRef => receiveConstRef
We best set breakpoints in the copy constructor and assignment operator of the Copy
class. If our program only calls emit sendConstRef(c)
, the breakpoints are not hit at all. So, no copies happen. Why?
The result is not really surprising, because this is exactly how passing arguments as const references in C++ works and because a direct signal-slot connection is nothing else but a chain of synchronous or direct C++ function calls.
Nevertheless, it is instructive to look at the chain of function calls executed when the sendConstRef
signal is emitted.
The meta-object code of steps 2, 3 and 4 – for marshalling the arguments of a signal, routing the emitted signal to the connected slots and de-marshalling the arguments for the slot, respectively – is written in such a way that no copying of the arguments occurs. This leaves us with two places, where copying of a Copy
object could potentially occur: when passing the Copy
object to the functions MainView::sendConstRef
or MainView::receiveConstRef
.
These two places are governed by standard C++ behaviour. Copying is not needed, because both functions take their arguments as const references. There are also no life-time issues for the Copy
object, because receiveConstRef
returns before the Copy
object goes out of scope at the end of sendConstRef
.
sendConstRef => receiveValue
Based on the detailed analysis in the last section, we can easily figure out that only one copy is needed in this scenario. When qt_static_meta_call
calls receiveValue(Copy c)
in step 4, the original Copy
object is passed by value and hence must be copied.
sendValue => receiveConstRef
One copy happens, when the Copy
object is passed by value to sendValue
by value.
sendValue => receiveValue

This is the worst case. Two copies happen, one when the Copy
object is passed to sendValue
by value and another one when the Copy
object is passed to receiveValue
by value.
Queued Connections
A queued signal-slot connection is nothing else but an asynchronous function call. Conceptually, the routing function QMetaObject::activate
does not call the slot directly any more, but creates a command object from the slot and its arguments and inserts this command object into the event queue. When it is the command object’s turn, the dispatcher of the event loop will remove the command object from the queue and execute it by calling the slot.
When QMetaObject::activate
creates the command object, it stores a copy of the Copy
object in the command object. Therefore, we have one extra copy for every signal-slot combination.
We must register the Copy
class with Qt’s meta-object system with the command qRegisterMetaType('Copy');
in order to make the routing of QMetaObject::activate
work. Any meta type is required to have a public default constructor, copy constructor and destructor. That’s why Copy
has a default constructor.
Queued connections do not only work for situations where the sender of the signal and the receiver of the signal are in the same thread, but also when the sender and receiver are in different threads. Even in a multi-threaded scenario, we should pass arguments to signals and slots by const reference to avoid unnecessary copying of the arguments. Qt makes sure that the arguments are copied before they cross any thread boundaries.
Conclusion
How Qt Signal And Slots Works
The following table summarises our results. The first line, for example, reads as follows: If the program passes the argument by const reference to the signal and also by const reference to the slot, there are no copies for a direct connection and one copy for a queued connection.
Qt Signal Slot Thread
Signal | Slot | Direct | Queued |
---|---|---|---|
const Copy& | const Copy& | 0 | 1 |
const Copy& | Copy | 1 | 2 |
Copy | const Copy& | 1 | 2 |
Copy | Copy | 2 | 3 |
The conclusion from the above results is that we should pass arguments to signals and slots by const reference and not by value. This advice is true for both direct and queued connections. Even if the sender of the signal and the receiver of the slot are in different threads, we should still pass arguments by const reference. Qt takes care of copying the arguments, before they cross the thread boundaries – and everything is fine.
By the way, it doesn’t matter whether we specify the argument in a connect call as const Copy&
or Copy
. Qt normalises the type to Copy
any way. This normalisation does not imply, however, that arguments of signals and slots are always copied – no matter whether they are passed by const reference or by value.